In today’s interconnected world, messaging platforms play a crucial role in communication. Telegram, a popular messaging app, provides a powerful API that allows developers to create Telegram bots and integrate them into their applications. In this blog post, we will explore how to integrate a Telegram bot into an Express application and send messages to Telegram using the Telegram Bot API.
Before diving into the integration process, make sure you have the following prerequisites:
- A Telegram account: Create an account on Telegram if you don’t already have one.
- Telegram Bot: Create a Telegram bot using the BotFather. This will provide you with an API token that you’ll use to authenticate your bot in the integration process.
- Node.js and npm: Ensure you have Node.js and npm (Node Package Manager) installed on your development machine.
Obtaining the API ID
To integrate a Telegram bot into your application, you need to obtain an API ID from Telegram. The API ID is a unique identifier associated with your application. Here’s how you can obtain the API ID:
Step 1: Create a Telegram Application:
- Visit the Telegram website (https://telegram.org) and sign in to your account.
- Go to the Telegram API development tools page (https://my.telegram.org/apps) and log in using your Telegram account credentials.
Step 2: Create a New Application:
- Click on the “API development tools” button. It will go to Create Application page.
- Fill in the required information, such as the name, platform, and description of your application.
- Specify the URL of your application’s website (if applicable).
Step 3: Obtain the API ID and Hash:
- Once your application is created, you will see the API ID and Hash on the application details page.
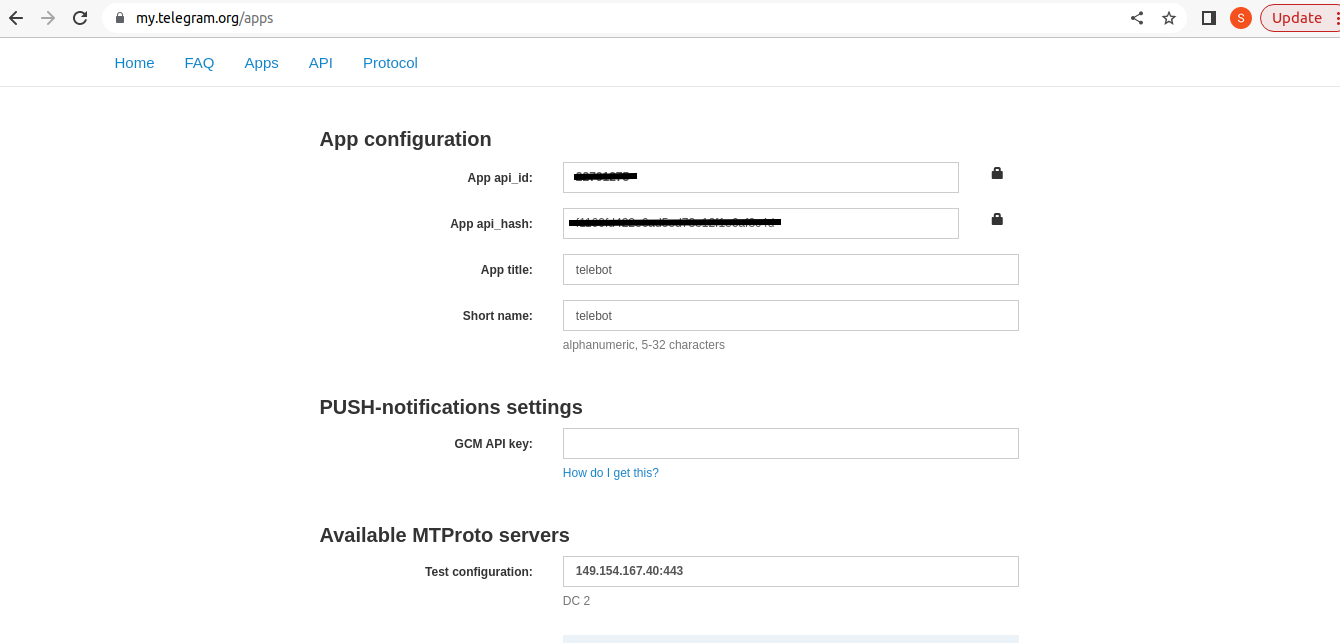
Go to your telegram application and type BotFather and go inside that. Create a new bot by typing /newbot and give appropriate name for your bot. Then BotFather will create a bot for you with Bot Token.
Creating the backend Application using express:
$ mkdir telebot
$ cd telebot
Install express using following command:
$ npm init -y
$ npm install express dotenv body-parser axios
Run the express app with command:
$ npm run start
Our application is running on port 5000.Here we are using ngrok.Start ngrok on port 5000 by typing the command:
ngrok http 5000
Now create a .env file and make sure ngrok is running on port 5000 and add the URL generated by ngrok to your .env file:
TOKEN="YOUR_BOT_TOKEN"
TELEGRAM_WEBHOOK_URL="NGROK_URL"
Create a .index.js file and give the following code there:
require("dotenv").config();
const express = require("express");
const axios = require("axios");
const bodyParser = require("body-parser");
const { TOKEN, TELEGRAM_WEBHOOK_URL } = process.env;
const TELEGRAM_API = `https://api.telegram.org/bot${TOKEN}`;
const URI = `/webhook/${TOKEN}`;
const WEBHOOK_URL = TELEGRAM_WEBHOOK_URL + URI;
const app = express();
app.use(bodyParser.json());
const init = async () => {
const res = await axios.get(`${TELEGRAM_API}/setWebhook?url=${WEBHOOK_URL}`);
};
app.post(URI, async (req, res) => {
const chatId = req.body.message?.chat.id;
const text = req.body.message?.text;
res.status(200).send("ok");
await axios.post(`${TELEGRAM_API}/sendMessage`, {
chat_id: chatId,
text: text,
});
return res.send();
});
app.listen(process.env.PORT || 5000, async () => {
console.log("🚀 app running on port", process.env.PORT || 5000);
await init();
});
When we send message to our telegram bot the telegram bot will give a response same as the message. So we successfully integrated our telegram bot with express Application.
Result of Telegram bot integration to Express Application
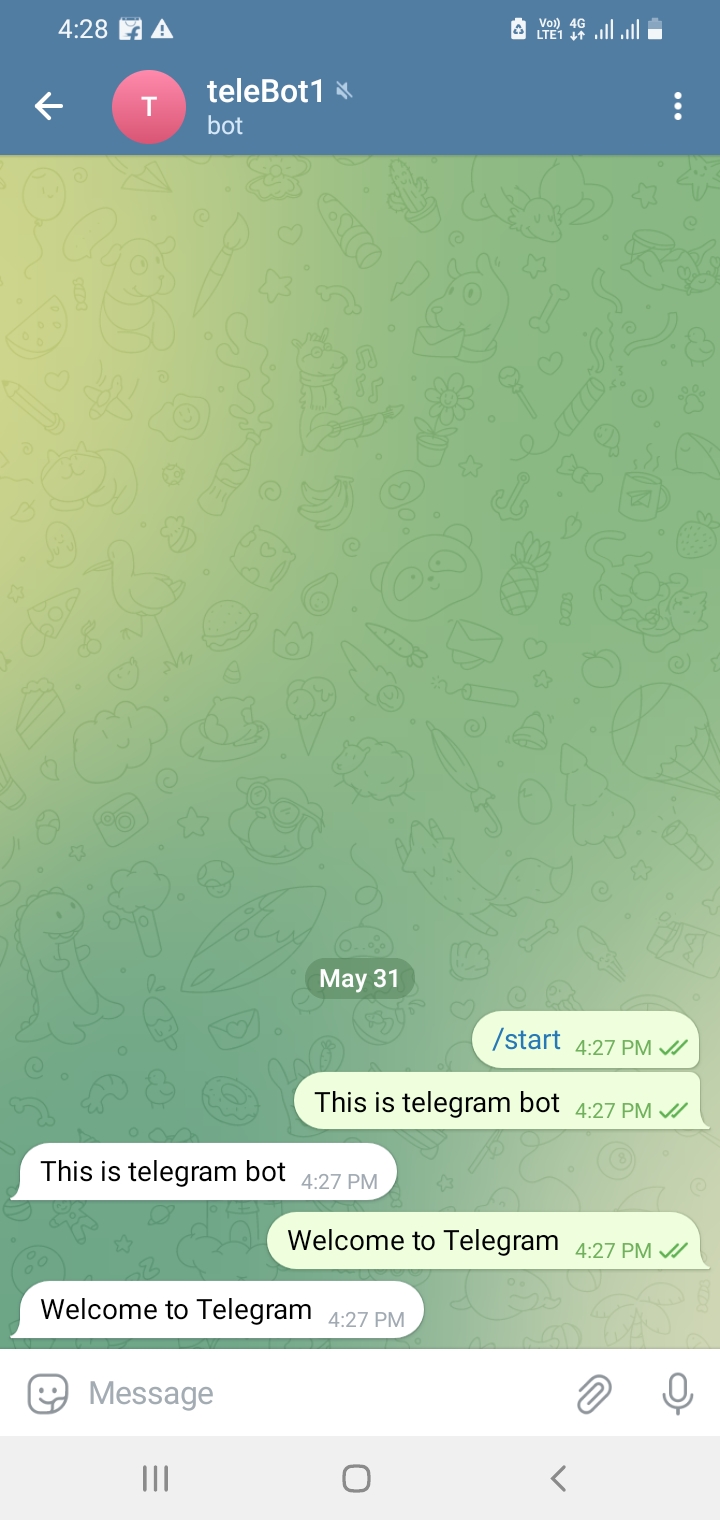
Conclusion
The Bot API allows developers to control Telegram Bots, for example receiving messages and replying to other users.