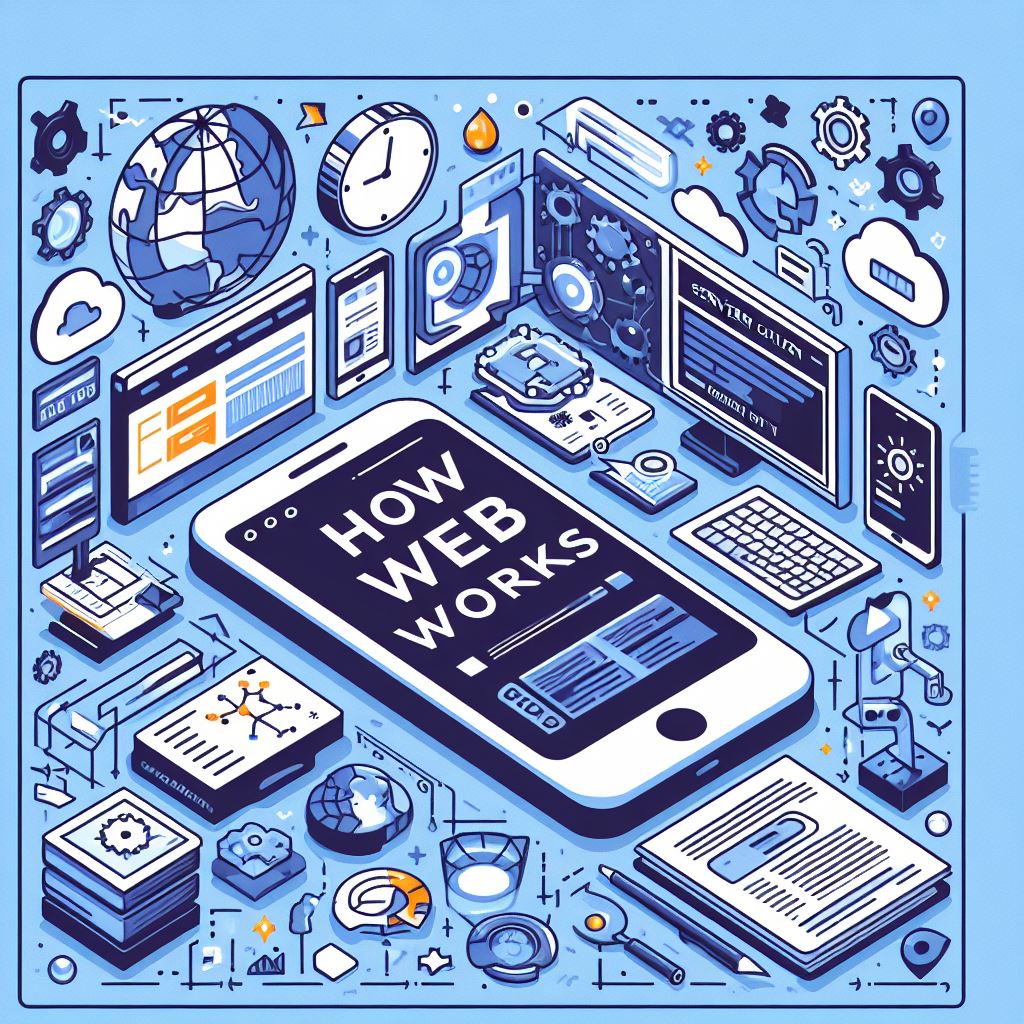
Welcome to the intricate world of web applications! In this blog, we’ll embark on a journey, exploring the inner workings of these digital marvels, from the fundamental building blocks like DNS and HTTP verbs to the elegant principles of RESTful APIs. And to make it even more exciting, we’ll be using Golang, a powerful and versatile language, as our guide.
DNS: The Domain Name Sorcerer
Imagine a phone book, but instead of names, it maps website addresses (URLs) to physical servers. That’s essentially what DNS or Domain Name System does. When you type in a website address, DNS translates it into an IP address, the unique identifier for the server hosting the website. This invisible magic behind the scenes ensures seamless access to your favorite websites, no matter where they reside in the digital world.
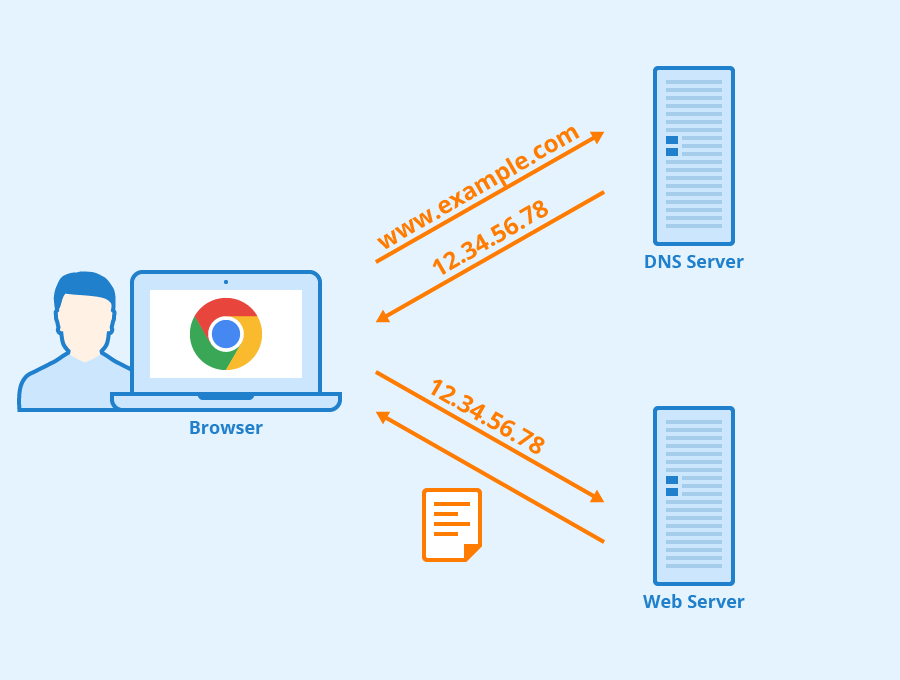
HTTP Verbs: The Language of Requests
Think of HTTP verbs as the different ways you can interact with a website. Get, Post, Put, Delete – these are just a few examples of these verbs, each with a specific purpose. Get retrieves information from a server, Post sends data to the server, Put updates existing data, and Delete removes data. Understanding these verbs is crucial for building web applications that communicate effectively with servers.
HTTP verbs, also known as HTTP methods, are actions used in Hypertext Transfer Protocol (HTTP) for interacting with resources located on a server. They form part of the request-response cycle in HTTP, allowing the client to perform various actions on the server. Here are some of the most commonly used HTTP verbs:
- GET: This method is used to retrieve data from the server. For example, when you visit a website, your browser sends a GET request to the server to fetch the web page.
- POST: This method is used to send data to the server. For example, when you fill out a form on a website and click on submit, your browser sends a POST request to the server with the form data.
- PUT: This method is used to update existing data on the server. It requires the client to send the entire updated entity, not just the changes.
- DELETE: This method is used to delete existing data on the server.
Understanding these HTTP verbs is crucial when building web applications, as they define the type of operation that is to be performed on the server-side data.
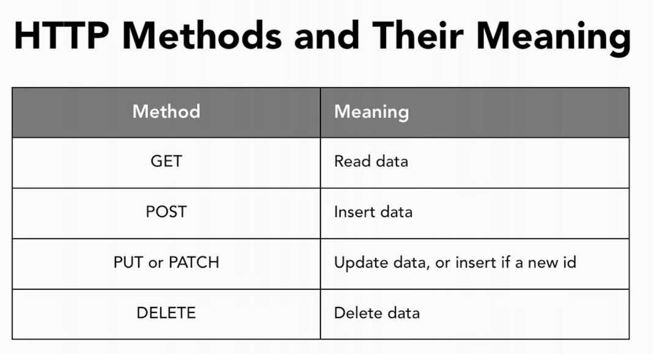
HTTP status codes are issued by a server in response to a client’s request made to the server. They represent whether a specific HTTP request has been completed. Below are some basic status codes that are commonly used:
- 200 OK: The request has succeeded. The information returned with the response is dependent on the method used in the request.
- 201 Created: The request has been fulfilled, resulting in the creation of a new resource.
- 204 No Content: The server successfully processed the request, but is not returning any content.
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 404 Not Found: The server can not find the requested resource. Links that lead to a 404 page are often called broken or dead links.
- 500 Internal Server Error: The server encountered an unexpected condition that prevented it from fulfilling the request.
RESTful APIs: The Architectural Elegance
APIs, or Application Programming Interfaces, are sets of rules and protocols that allow different software applications to communicate with each other. They define the methods and data formats that a program can use to communicate with other programs or components. APIs are used in a wide variety of contexts, from web development to operating systems, and allow for more complex and dynamic software applications.
REST, or Representational State Transfer, defines a set of principles for designing web APIs. It emphasizes resource-oriented architecture, using verbs like GET and POST to manipulate specific resources (e.g., user data, product information). This approach leads to clean, predictable, and scalable APIs that are easy to understand and use.
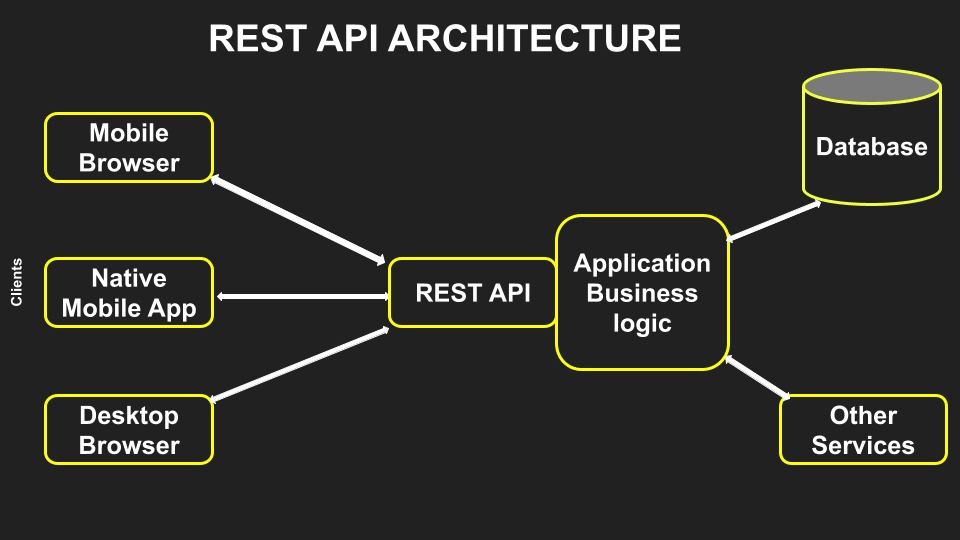
I think you have a basic understanding of the DNS, HTTP Verbs, and Restful APIs.
Let’s get our hands dirty! We’ll build a simple Golang API that exposes a “users” resource. Users can be retrieved using GET, created using POST, updated using PUT , and deleted using DELETE
. This example will showcase how Golang handles requests and responses, demonstrating RESTful principles in action. Before that a brief about the Golang
Go, often nicknamed “Golang,” is a powerful and versatile programming language that makes web development a breeze. Imagine a language that combines the simplicity and beauty of Python with the speed and concurrency of C. That’s Go! Its built-in features like net/http
handle communication with websites effortlessly, while its compact syntax and focus on clarity make writing and reading the code a joy. Whether you’re a seasoned developer seeking a faster, more efficient tool or a curious beginner looking for a friendly entry point, Go is worth the journey. Its rapidly growing community and vast ecosystem of libraries ensure you’ll have the support and resources to build your dream web applications. So, grab your Golang toolkit and unleash your inner architect – the web awaits!
Before we dive into code, let’s introduce Gin! and GORM Think of it as your trusty steed in the API development landscape. Gin gallops ahead with features like:
- Effortless Routing: Define precise paths for your API endpoints with just a few lines of code.
- Clean Middle-ware: Inject functionalities like authentication, logging, and error handling seamlessly into your API workflow.
- JSON Simplicity: Encode and decode data effortlessly between your application and the outside world.
- Concise Syntax: Write clean, readable code that focuses on logic, not framework boilerplate.
GORM is a developer-friendly ORM library for handling relational databases. It stands for “Go Object-Relational Mapping” and it’s written in the Go language. It abstracts the complexities involved in handling SQL queries and allows you to interact with the database using Go structs.
GORM supports a wide range of databases like MySQL, PostgreSQL, SQLite, and SQL Server. It offers a simple and intuitive API for handling database operations like Create, Read, Update, and Delete (CRUD). It also supports advanced functionalities like Associations, Hooks, Transactions, Migrations, etc.
For example, if you have a User
struct in your Go program, you can easily save a user, fetch users, update user information, and delete a user from the database without writing any SQL query. GORM takes care of translating your Go code into SQL queries under the hood.
In addition to these, GORM also includes features such as Preloading (eager loading), Transactions, Migrations, SQL Builder, Query Builder, and more. It also allows you to write raw SQL if you need it for complex queries. Overall, GORM is a rich, idiomatic, and developer-friendly library that makes it easy to handle database operations in Go applications.
Now, let’s put our learning into action! We’ll build a simple user API using Golang and Gin, showcasing how this dynamic duo simplifies API development. We’ll expose endpoints to:
- Retrieve all users: Imagine a
GET /users
request, instantly fetching a list of users from your database. - Create a new user: With a
POST /users
request, send user data and watch as Gin and Golang work their magic to add a new member to your user community. - Update existing users: Want to change a user’s name or email? A
PUT /users/:id
request and some updated data are all it takes. - Delete users: Need to remove a user? Simply send a
DELETE /users/:id
request, and watch as the user gracefully exits your platform.
Code with Clarity: Throughout this journey, we’ll explore Golang and Gin code snippets, focusing on clarity and understanding. You’ll see how Gin’s routing definitions (r.GET), context access (c.Param), and JSON handling (think c.BindJSON and c.JSON) make building powerful APIs a breeze.
- Set up the project:
Create a new Go project directory and initialize a Go module:
mkdir golang-api
- cd golang-api
- go mod init golang-api
This command initializes a new Go module for your project. It creates a go.mod
file that tracks the dependencies of your project. The module name is set to golang-api
.
Install the Gin package for crafting well-structured RESTful APIs, enabling seamless communication between your back-end and front-end applications.
Gin is a high-performance micro-framework that delivers a radical increase in productivity for writing web applications in Go. It does this by leaning on net/HTTP package primitives, making it quick and easy to get up and running with a new web application.
Gin provides a simple, expressive API to make routing and middleware easy to use. It also has added conveniences like JSON validation and rendering, making it a robust foundation for your web applications.
Developers enjoy using Gin because it’s easy to learn and intuitive to work with, and it aligns well with Go’s philosophy of simplicity and straightforwardness. Additionally, being extremely lightweight with a minimalistic setup, it allows developers to focus more on writing business logic rather than boilerplate code.
go get -u github.com/gin-gonic/gin
Create a main.go file and define handlers for different HTTP methods:
package main
import (
"github.com/gin-gonic/gin"
"gorm.io/driver/postgres"
"gorm.io/gorm"
)
type User struct {
ID uint `json:"id" gorm:"primaryKey"`
Name string `json:"name"`
Email string `json:"email"`
}
func main() {
// Connect to database
db, err := connectDB()
if err != nil {
panic("failed to connect to database")
}
// Migrate the User model
db.AutoMigrate(&User{})
r := gin.Default()
// Define API endpoints using separate functions
r.GET("/users", getUsersHandler(db))
r.POST("/users", createUserHandler(db))
r.PUT("/users/:id", updateUserHandler(db))
r.DELETE("/users/:id", deleteUserHandler(db))
r.Run() // Start the Gin server
}
func connectDB() (*gorm.DB, error) {
return gorm.Open(postgres.Open("host=localhost user=your_user dbname=your_db password=your_password"), &gorm.Config{})
}
func getUsersHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
var users []User
db.Find(&users)
c.JSON(200, users)
}
}
func createUserHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
var newUser User
if err := c.BindJSON(&newUser); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
db.Create(&newUser)
c.JSON(201, newUser)
}
}
func updateUserHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
id := c.Param("id")
var updatedUser User
if err := c.BindJSON(&updatedUser); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
db.First(&User{}, id).Updates(&updatedUser)
c.JSON(200, updatedUser)
}
}
func deleteUserHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
id := c.Param("id")
db.Delete(&User{}, id)
c.JSON(204, gin.H{})
}
}
Let’s break down the code one by one
- Define a simple User struct:
type User struct {
ID uint json:"id" gorm:"primaryKey"
Name string json:"name"
Email string json:"email"
}
In GoLang, a struct (short for structure) is a composite data type that groups variables of different types into a single unit. These variables are known as fields, and they each have their own name and type. A struct can be used to represent a wide range of data structures and is particularly useful for grouping related data together, making it easier to handle and manipulate.
For example, a User
struct might include fields for a user’s ID, name, and email. These fields would be defined like this:
type User struct {
ID uint `json:"id" gorm:"primaryKey"`
Name string `json:"name"`
Email string `json:"email"`
}
In this example, ID is an unsigned integer, while Name and Email are strings. The json and gorm tags define how the fields are treated when the struct is used with JSON encoding/decoding and the GORM database library, respectively.
- In the main file, we could define the main function and the function to establish the database (DB) connection
func main() {
// Connect to database
db, err := connectDB()
if err != nil {
panic("failed to connect to database")
}
// Migrate the User model
db.AutoMigrate(&User{})
r := gin.Default()
// Define API endpoints using separate functions
r.GET("/users", getUsersHandler(db))
r.POST("/users", createUserHandler(db))
r.PUT("/users/:id", updateUserHandler(db))
r.DELETE("/users/:id", deleteUserHandler(db))
r.Run() // Start the Gin server
}
func connectDB() (*gorm.DB, error) {
return gorm.Open(postgres.Open("host=localhost user=your_user dbname=your_db password=your_password"), &gorm.Config{})
}
This code sets up a simple user API using Gin and GORM. It first connects to your PostgreSQL database and ensures the User model schema is in place. Then, it creates a Gin server and defines four API endpoints: one to fetch all users, another to create new users, a third to update existing users, and finally, one to delete a user. Each endpoint uses a dedicated handler function for cleaner, organized code. By running the server, you make your API accessible, ready to process user-related requests, and handle the corresponding database operations.
The db.AutoMigrate function in GORM is used to automatically migrate your schema, to keep it in sync with your Go structs. It will ONLY create tables, missing columns, and missing indexes, and WON’T change existing column types or delete unused columns to protect your data.
func getUsersHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
var users []User
db.Find(&users)
c.JSON(200, users)
}
}
This code outlines a function getUsersHandler serving as a handler for a route in a Gin web server. Upon receiving a request at this route, the function retrieves all users from the database utilising GORM, bundling them into a “User” structure array. It then returns a JSON response to the client, which includes the extracted user data, signaling a successful operation with a 200 status code. Essentially, this code enables the retrieval of all users within the application.
func createUserHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
var newUser User
if err := c.BindJSON(&newUser); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
db.Create(&newUser)
c.JSON(201, newUser)
}
The code block createUserHandlers
defines a function that handles the creation of a new user in a Gin web application. It accepts a database connection and returns a handler function that’s executed when a request matches the specific route. First, it attempts to bind incoming JSON data to a new User struct. If there’s an error during this process, it sends a JSON response with a 400 status code (Bad Request) and an error message. If successful, it proceeds to create the new user in the database using GORM. Finally, it sends a JSON response with a 201 status code (Created) and the newly created user data, confirming successful user creation.
func updateUserHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
id := c.Param("id")
var updatedUser User
if err := c.BindJSON(&updatedUser); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
db.First(&User{}, id).Updates(&updatedUser)
c.JSON(200, updatedUser)
}
}
This code block updateUserHandler
outlines a function for updating user information in a Gin web application. The function takes a database connection and returns a handler function which is triggered when a request meets the specific route. Initially, it obtains the user’s ID from the request’s parameters and tries to bind the incoming JSON data to an “updatedUser” struct. If there are any errors during the binding process, a JSON response is sent with a 400 status code (Bad Request) and an error message. On successful binding, it locates the user in the database using GORM and applies the updates to the user data. Finally, it sends a JSON response with a 200 status code (OK) and the updated user data, indicating a successful update.
func deleteUserHandler(db *gorm.DB) gin.HandlerFunc {
return func(c *gin.Context) {
id := c.Param("id")
db.Delete(&User{}, id)
c.JSON(204, gin.H{})
}
}
This code block deleteUserHandler
defines a function that handles the deletion of a user in a Gin web application. It accepts a database connection and returns a handler function that’s executed when a request hits a specific route. It first extracts the user’s ID from the request’s parameters and then proceeds to delete the user with that ID from the database using GORM. Finally, it sends a JSON response with a status code of 204 (No Content), indicating that the deletion was successful but without returning any content in the response body.
To run the project
Navigate to the project directory: Open a terminal and navigate to the project’s root directory.
cd golang-api
Execute the main file: Run the command go run main.go
to start the API server.
Conclusion
The web is a complex system, but understanding its core components empowers you to navigate its depths. Websites? Think giant libraries with hidden treasure – DNS is the map, leading your browser to the right one. HTTP verbs? Courier bots, carry your requests (wanting that recipe) and responses (the recipe itself) between the browser and website. Building these libraries? Code! Languages like Golang are the building blocks, and frameworks like Gin are prefabricated modules, making development faster. Finally, those treasures? They’re stored in encrypted vaults called databases, accessible through tools like GORM. So, there you have it! The web isn’t magic, it’s intricate technology. Now equipped with this knowledge, you can appreciate the symphony of protocols and programs powering every click and scroll. Ready to dive deeper? The web awaits your exploration!