What is an image placeholder?
Having too many images in your app or images that are very large and load slowly can negatively impact page speed and user experience. In fact, images are probably one of the most significant contributors to your app’s speed.
Instead of reducing the number of images in an app, we can use placeholders for some of them, thereby reducing the number of image files on initial load.
An image placeholder is a dummy image that the browser can load initially to represent the actual image until the true image has been downloaded. Placeholders can enhance page speed because their file size is tiny compared to that of the actual image they represent. Image placeholders can also prevent webpage layout shifts from occurring.
The placeholder could be a thumbnail of the image, an empty gray box, an img
element with a border or background color, or even the dimensions of the image (if fixed) or the image’s alt text. However, none of these options are pretty, and all of them will make your webpages look boring.
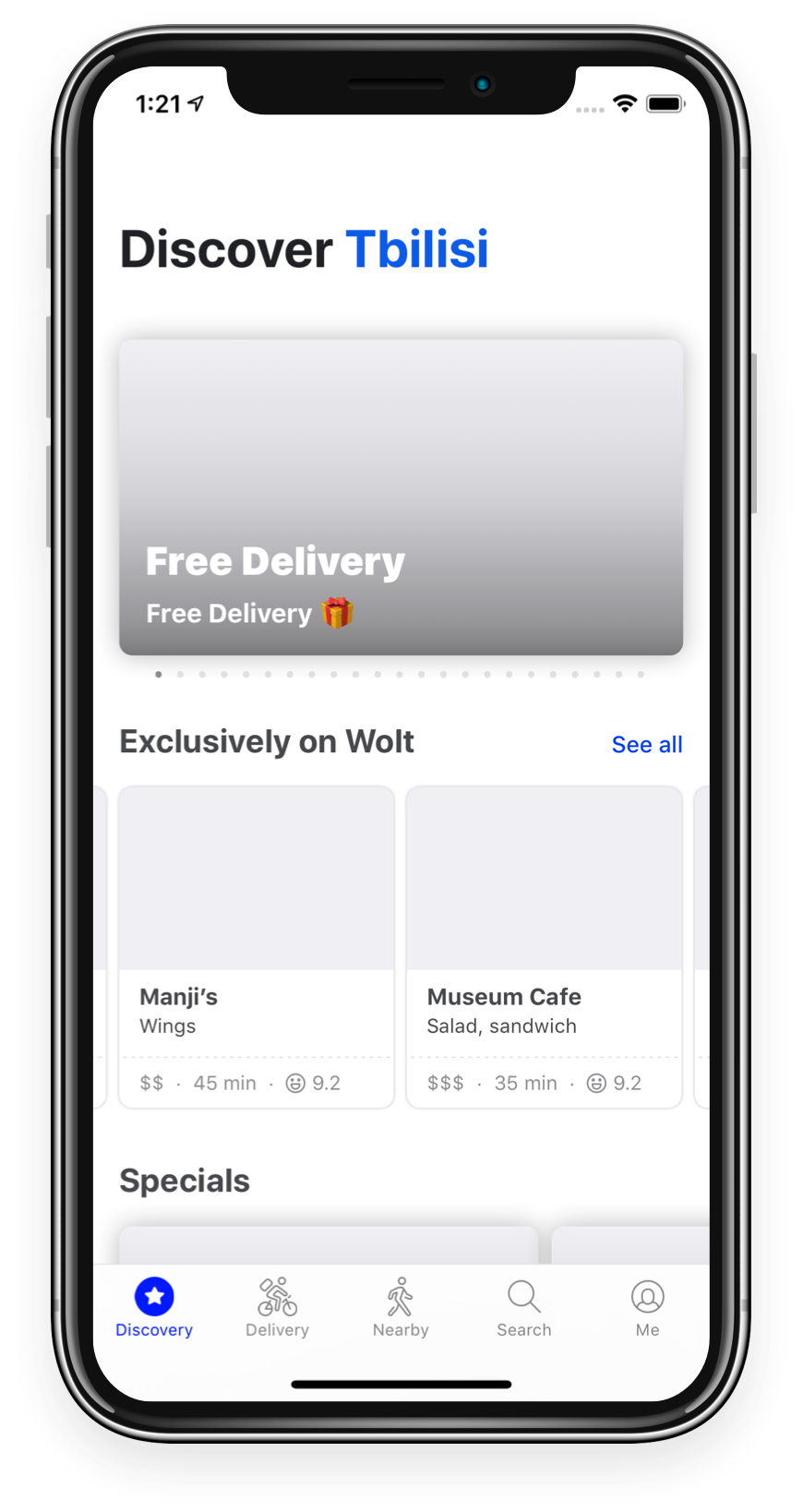
Now, let’s consider more aesthetic option for the placeholder.
What is BlurHash?
To make the image placeholder more visually appealing, we can use the BlurHash method to create a blurred version of the image, resulting in a more visually appealing design.
BlurHash is a technique used to encode an image into a short string of text or code. This code can then be used to display a low-quality, blurred version of the image before the actual image loads. The idea behind BlurHash is to give users an idea of what the image will look like before it fully loads, without sacrificing performance.
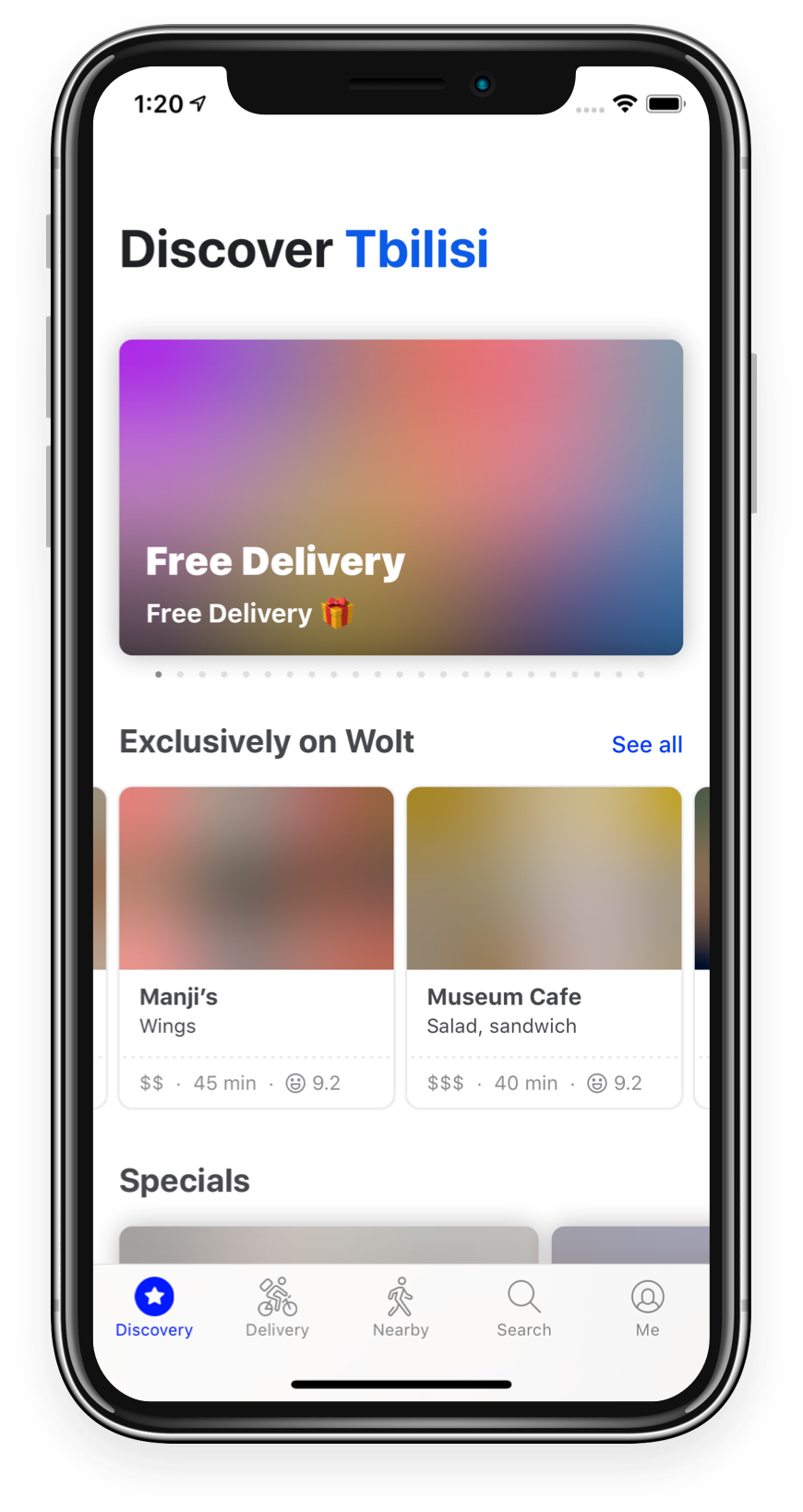
Using this BlurHash string as your image placeholder makes your webpage more visually appealing and also reduces the page speed or initial load of your webpage.
Real time application that uses blur hash is Unsplash.
How does BlurHash work?
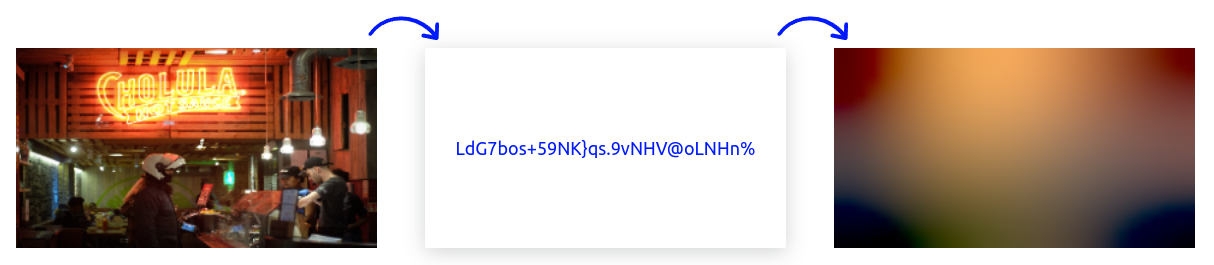
The BlurHash algorithm analyzes an image and generates a string that serves as the image’s placeholder.
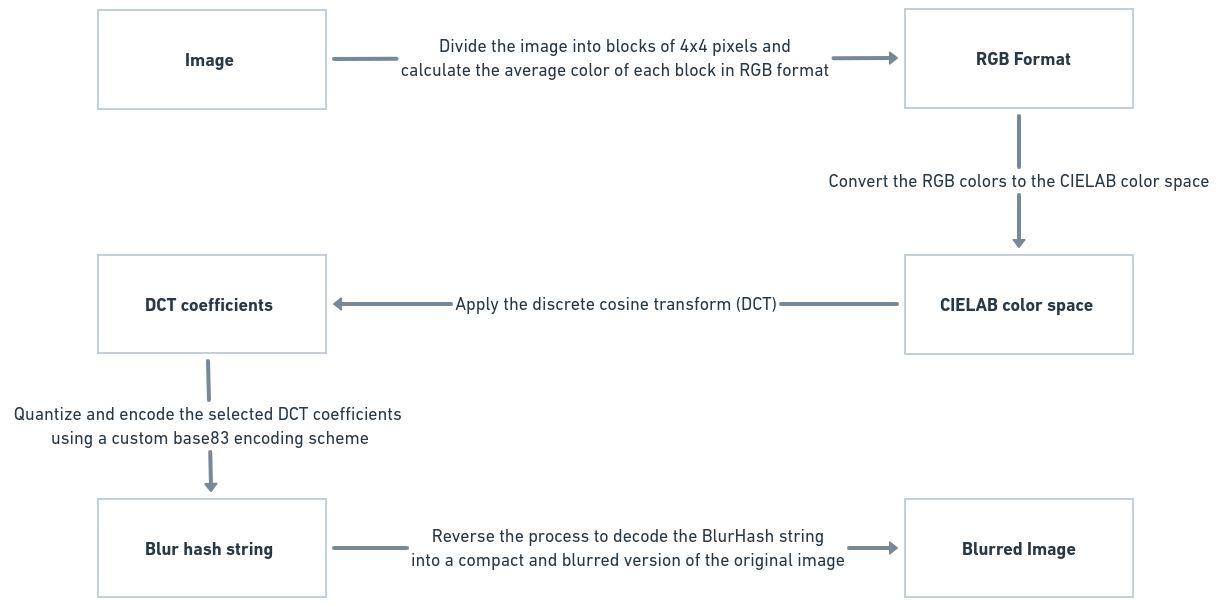
This process is usually done at the backend of your service, where the image URL is stored, so you can keep the BlurHash string alongside the image URL. The BlurHash string and the image URL are sent to the client together.
The BlurHash string is short enough to quickly fit into whatever data type you prefer. For example, it’s easy to include it as a field in a JSON object.
To display a BlurHash placeholder image while the actual picture loads over the network, your client first takes the BlurHash string and decodes it into an image while its full-size version loads in the background.
How do you implement BlurHash in a your application?
The best part about BlurHash is that you can easily import it to your favorite programming language or platform of choice.
Here’s an example using node.js and React.
Generate BlurHash string using node.js application
Use the Javascript decoder and encoder for BlurHash algorithm,
npm install --save blurhash
Uasge,
import { encode } from "blurhash";
const loadImage = async src =>
new Promise((resolve, reject) => {
const img = new Image();
img.onload = () => resolve(img);
img.onerror = (...args) => reject(args);
img.src = src;
});
const getImageData = image => {
const canvas = document.createElement("canvas");
canvas.width = image.width;
canvas.height = image.height;
const context = canvas.getContext("2d");
context.drawImage(image, 0, 0);
return context.getImageData(0, 0, image.width, image.height);
};
const encodeImageToBlurhash = async imageUrl => {
const image = await loadImage(imageUrl);
const imageData = getImageData(image);
return encode(imageData.data, imageData.width, imageData.height, 4, 4);
};
The encodeImageToBlurhash
will retun an encoded string when provided with an actual image url. Store this string in your database along with actual image url.
{
src: "<https://images.unsplash.com/photo-1574641264510-d656942d6380>",
blurhash: “UdG7bos+59NK}qs.9vNHV@oLNHn%Iqa}t6t6”
}
Adding the BlurHash URL to a React application
React components for using the blurhash algorithm,
npm install --save blurhash react-blurhash
Usage,
import { Suspense } from 'react';
import { Blurhash } from 'react-blurhash';
const ImageComponent = ({ image }) => {
return (
<div>
<Suspense
fallback={
<Blurhash
hash={image?.blurhash}
width={400}
height={300}
resolutionX={32}
resolutionY={32}
punch={1}
/>
}
>
<img src={image?.url} alt='Some image' />
</Suspense>
</div>
);
};
export default ImageComponent;
Props
name | description |
---|---|
hash (string) | The encoded blurhash string. |
width (int | string) |
height (int | string) |
resolutionX (int) | The X-axis resolution in which the decoded image will be rendered at. Recommended min. 32px. Large sizes (>128px) will greatly decrease rendering performance. (Default: 32) |
resolutionY (int) | The Y-axis resolution in which the decoded image will be rendered at. Recommended min. 32px. Large sizes (>128px) will greatly decrease rendering performance. (Default: 32) |
punch (int) | Controls the “punch” value (~contrast) of the blurhash decoding algorithm. (Default: 1) |
You can find a blurhash playground here react-blurhash.
Conclusion
In this article, we demonstrated what is BlurHash and how to use BlurHash to generate placeholder images for a React application. This strategy results in a visually pleasing webpage, prevents layout shifts by ensuring that page contents retain their position, and improves the app’s page load speed. In turn, this improves the performance of you React application.