The Error Widget is typically used to display an error message when the app encounters an exception or a problem that prevents it from rendering correctly. This could be caused by a variety of factors, such as an issue with the app’s code, a problem with the device’s hardware or software, or a problem with the network connection. When an error occurs, the ErrorWidget is automatically displayed, providing the user with a clear indication that something went wrong and that the app is unable to continue.
You can easily implement an Error Widget by adding ErrorWidget.builder to the main() function like this:
void main() {
ErrorWidget.builder = (FlutterErrorDetails details) {
return <your widget>;
};
return runApp(MyApp());
}
How to use Error Widget Builder in Flutter
Just for an example I am throwing an error from initState() method as below:
void main() {
ErrorWidget.builder = (FlutterErrorDetails details) {
return CustomErrorScreen(details: details);
};
runApp(MyApp());
}
class MyApp extends StatefulWidget {
const MyApp({Key? key}) : super(key: key);
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: HomePage(),
);
}
@override
void initState() {
// TODO: implement initState
super.initState();
throw ("Error thrown from initState()");
}
}
One of the key features of the ErrorWidget is that it can be customized to display a custom error message or icon. This allows developers to provide more detailed information about the error and provide guidance on how the user can resolve the problem.
class CustomErrorScreen extends StatelessWidget {
const CustomErrorScreen({Key? key, required this.details}) : super(key: key);
final FlutterErrorDetails details;
@override
Widget build(BuildContext context) {
return Material(
color: Colors.white,
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Image.asset(errorImage),
const Text(
"Error",
textDirection: TextDirection.ltr,
style: TextStyle(
fontSize: 50,
fontWeight: FontWeight.w700,
),
),
const SizedBox(
height: 20,
),
Text(
"${details.exception} ",
textDirection: TextDirection.ltr,
style: const TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
),
),
],
),
),
);
}
}
Result of ErrorWidget for Error handling in a flutter
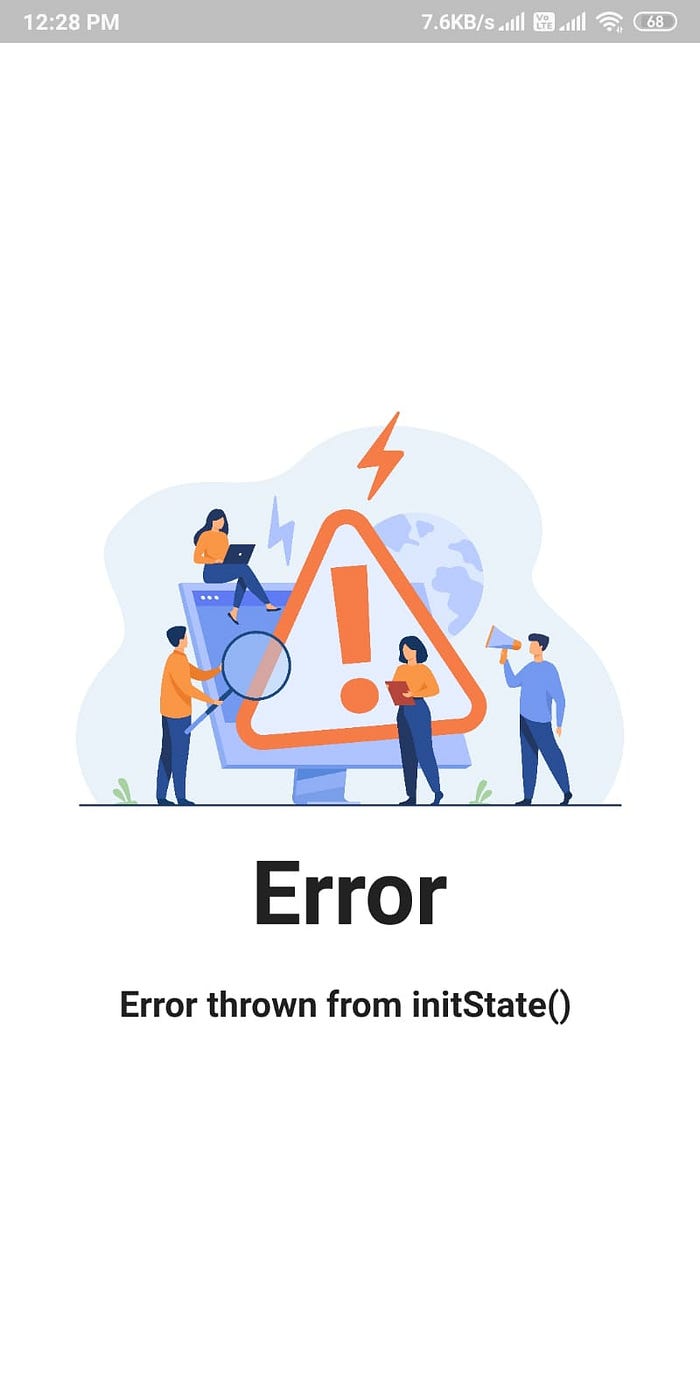
Conclusion
In conclusion, the ErrorWidget is an important built-in widget in Flutter that plays a key role in handling errors and improving the user experience of your app.
References
https://api.flutter.dev/flutter/widgets/ErrorWidget-class.html